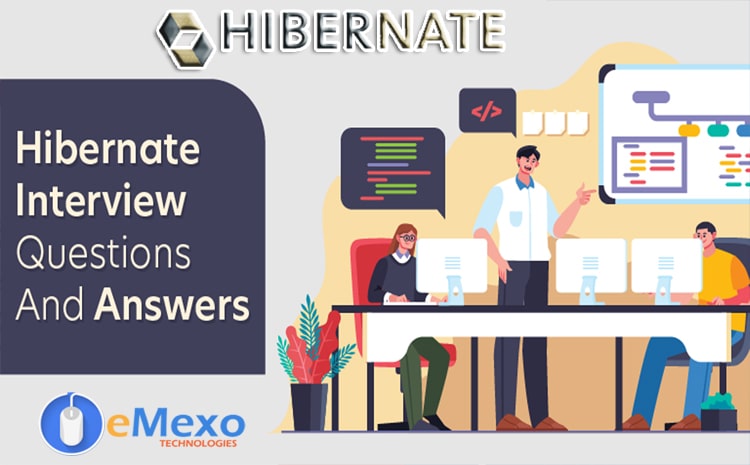
As a widely used ORM tool, In interviews students are asked questions about Hibernate. So that eMexo Technologies provides an basic list of top 21 Hibernate interview questions and answers for freshers and professionals.
1. What is Hibernate Framework?
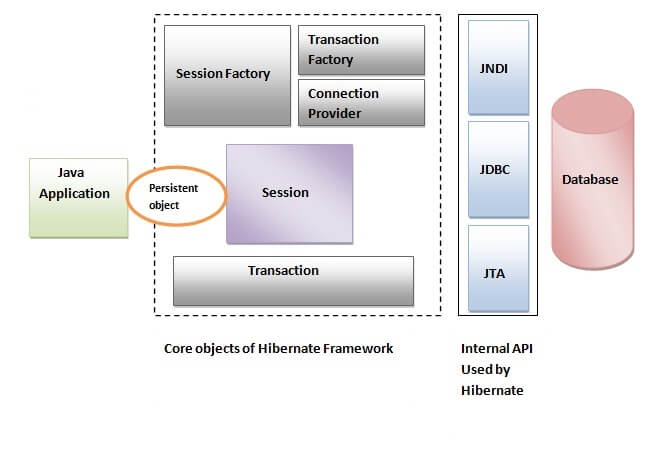
- Object-relational mapping or ORM is a programming technique for mapping application domain model objects to relational database tables.
- Hibernate is a Java-based ORM tool that provides a framework for mapping application domain objects to relational database tables and vice versa.
- Hibernate provides the reference implementation of the JavaPersistence API and is ideal as an ORM tool with the benefits of loose coupling.
- You can use the Hibernate persistence API for CRUD operations.
- The Hibernate framework provides the ability to map plain old Java objects to traditional database tables using JPA annotations and XML-based configurations.
2. What is Java Persistence API (JPA)?
Java Persistence API (JPA) gives specifications for handling the relational data in applications.
3. What is Hibernate SessionFactory and how to configure it?
- SessionFactory is a factory class used to get a session object.
- SessionFactory is responsible for reading hibernate configuration parameters, connecting to the database, and providing session objects.
- Normally, an application has a single SessionFactory instance, and the thread that processes the client request gets the Session instance from that factory.
- The internal state of SessionFactory is immutable. Once created, this internal state is set.
- This internal state contains all object / relational mapping metadata.
- SessionFactory also provides methods to get class metadata and statistical instances to get query execution statistics, second level cache details, and more.
- The internal state of SessionFactory is immutable, so it is thread-safe. Multiple threads can access at the same time to get a session instance.
4. What is Hibernate Session and how to get it?
- Hibernate Session is the interface between the Java application layer and Hibernate.
- This is the core interface used to perform database operations.
- The session life cycle is associated with the start and end of a transaction.
- Sessions provide methods for performing create, read, update, and delete operations on persistent objects.
- You can execute HQL and native SQL queries and use session objects to create conditions.
5. What is difference between openSession and getCurrentSession?
The Hibernate SessionFactory’s getCurrentSession () method returns a session bound to the context. However, for this to work, it must be configured with a hibernate configuration file. This session object belongs to the Hibernate context and does not need to be closed. This session object is closed when the session factory is closed.
<property name="hibernate.current_session_context_class">thread</property> The Hibernate SessionFactory's openSession () method always opens a new session. This session object should be closed when all database operations are complete. In a multithreaded environment, you need to open a new session for each request.
6. What is difference between Hibernate Session get() and load() method?
Hibernate sessions provide various methods for loading data from the database. get and load are the most commonly used methods, and although they look similar at first glance, there are some differences.
get () loads the data as soon as it is called, while load () returns a proxy object and loads the data only when it is really needed. Therefore, load () is great because it supports lazy loading.
load () throws an exception if no data is found, so it should only be used if the data is known to exist.
If you want to verify that the data exists in the database, you need to use get ().
7. What is hibernate caching? Explain Hibernate first level cache?
As the name implies, Hibernate stores query data to speed up your application. Proper use of Hibernate Cache can be very helpful in accelerating application performance. The idea behind the cache is to reduce the number of database queries, thereby reducing application throughput time. Hibernate FirstLevelCache is associated with the Session object. Hibernate First Level Cache is enabled by default and cannot be disabled. However, Hibernate provides methods that allow you to remove selected objects from the cache or completely empty the cache. Objects cached in a session are not visible to other sessions, and all cached objects are lost when the session is closed.
8. What are different states of an entity bean?
An entity bean instance can be in one of three states.
- Temporary: If an object is not persisted or associated with a session, it will be in a temporary state. Temporary instances can be persisted by calling save (), persist (), or saveOrUpdate (). Persistent instances can be temporary by calling delete ().
- Persistent: If an object is associated with a unique session, it will be in a persistent state. All instances returned by the get () or load () methods are persistent.
- Detach: If an object was previously persistent but was not associated with a session, it will be in a detached state. Disconnected instances can be persisted by calling update (), saveOrUpdate (), lock (), or replicate (). The state of a temporary or disconnected instance can also be persisted as a new persistent instance by calling merge ().
9. How to implement Joins in Hibernate?
There are several ways to implement idle joins.
Use one-to-one, one-to-many, and other associations.
Using JOINs in HQL queries. There is another format “JoinFetch” that loads related data at once. There is no lazy loading.
You can use the join keyword to trigger a native SQL query.
10. What is HQL and what are it’s benefits?
The Hibernate Framework has the Hibernate Query Language (HQL), a powerful object-oriented query language. It’s very similar to SQL, but it’s closer to object-oriented programming because it uses objects instead of table names.
The Hibernate query language is case insensitive except for Java classes and variable names. So SeLeCT is sELEct the same as SELECT, but com.journaldev.model.Employee is not the same as com.journaldev.model.EMPLOYEE.
HQL queries are cached, but this should be avoided as much as possible. Otherwise, you will have to worry about the association. However, because of its object-oriented approach, this is a better choice than native SQL queries.
11. What is Query Cache in Hibernate?
Hibernate implements a cache region for queries resultset that integrates closely with the hibernate second-level cache.This is an optional feature and requires additional steps in code. This is only useful for queries that are run frequently with the same parameters. First of all we need to configure below property in hibernate configuration file.
<property name = “hibernate.cache.use_query_cache”>true </property>
And in code, we need to use setCacheable(true) method of Query, quick example looks like below.
Query query = session.createQuery(“from Employee”); query.setCacheable(true); query.setCacheRegion(“ALL_EMP”); |
12. Can we execute native sql query in hibernate?
Hibernate provides the ability to execute native SQL queries using SQLQuery objects.
However, this is not the recommended approach as it loses the benefits associated with Hibernate mapping and first level Hibernate caching in normal scenarios.
13. What is the benefit of native sql query support in hibernate?
Native SQL queries are useful for executing database-specific queries that are not supported by the Hibernate API such as query hints or the CONNECT keyword in Oracle Database.
14. What is Named SQL Query?
Hibernate provides named queries that you can define in one place and use anywhere in your code. You can create both HQL and native SQL named queries.
Hibernate named queries can be defined in the Hibernate map file or using the @NamedQuery and @NamedNativeQueryJPA annotations.
15. What are the benefits of Named SQL Query?
Hibernate Named Query helps you group queries into one central location instead of keeping them distributed throughout your code.
The Hibernate named query syntax is checked when building the HibernateSessionFactory. This causes the application to fail immediately if an error occurs in the named query.
Hibernate Named Query is global. That is, once defined, it can be used throughout the application.
However, one of the main drawbacks of named queries is that they are difficult to debug because you have to find the defined location.
16. What is the benefit of Hibernate Criteria API?
Hibernate provides a more object-oriented Criteria API for querying the database and getting the results. You cannot use conditions to update or delete queries or DDL statements. It is only used to get results from the database using a more object-oriented approach.
Some of the most common uses of the Criteria API are:
- TheCriteriaAPI provides projections that can be used for aggregate functions such as sum (), min (), max ().
- You can use the Criteria API with ProjectionList to get only the selected columns.
- The Criteria API can be used in join queries by joining multiple tables.
- Useful methods are createAlias (), setFetchMode (), and setProjection ().
- You can use the Criteria API to get conditional results.
- A convenient method is add (), which allows you to add constraints.
- The Criteria API provides an addOrder () method that you can use to order the results.
17. What is Hibernate Proxy and how it helps in lazy loading?
Hibernate uses proxy objects to support lazy loading. Basically, when loading data from a table, Hibernate does not load all the associated objects. If you reference a child or search object via the getter method and the associated entity is not in the session cache, the proxy code goes to the database and loads the associated object. Use javassist to effectively and dynamically generate child implementations of entity objects.
18. How to implement relationships in hibernate?
You can easily implement one-to-one, one-to-many, and many-to-many relationships at hibernate. This can be done with both JPA annotations and XML-based configurations.
19. How transaction management works in Hibernate?
- Transaction management is very easy when idle, as most non-transactional operations are not allowed.
- Therefore, after getting a session from SessionFactory, you can call session beginTransaction () to start a transaction.
- This method returns a transaction reference that can be used later to commit or roll back the transaction.
- All hibernate transaction management is superior to JDBC transaction management because it does not have to rely on rollback exceptions.
- The exception thrown by the session method automatically rolls back the transaction.
20. What is cascading and what are different types of cascading?
When there is a relationship between entities, we need to define how different operations affect other entities. This is done by cascading and there are different types.
This is a simple example of using a cascade between a primary and a secondary entities.
import org.hibernate.annotations.Cascade; @Entity @Table(name = “EMPLOYEE”) public class Employee { @OneToOne(mappedBy = “employee”) @Cascade(value = org.hibernate.annotations.CascadeType.ALL) private Address address; } |
Note that Hibernate CascadeType enum constants are little bit different from JPA javax.persistence.CascadeType, so we need to use the Hibernate CascadeType and Cascade annotations for mappings, as shown in above example.
Commonly used cascading types as defined in CascadeType enum are:
None: No Cascading, it’s not a type but when we don’t define any cascading then no operations in parent affects the child.
ALL: Cascades save, delete, update, evict, lock, replicate, merge, persist. Basically everything
SAVE_UPDATE: Cascades save and update, available only in hibernate.
DELETE: Corresponds to the Hibernate native DELETE action, only in hibernate.
DETATCH, MERGE, PERSIST, REFRESH and REMOVE – for similar operations
LOCK: Corresponds to the Hibernate native LOCK action.
REPLICATE: Corresponds to the Hibernate native REPLICATE action.
21. Which design patterns are used in Hibernate framework?
Some of the design patterns used by the Hibernate Framework are:
- Domain Model Pattern-An object model for a domain that contains both behavior and data.
- Data Mapper-A mapper layer that moves data between objects and databases while maintaining independence from each other and from the mapper itself.
- Proxy pattern for Lazy loading
- Factory pattern in Session Factory