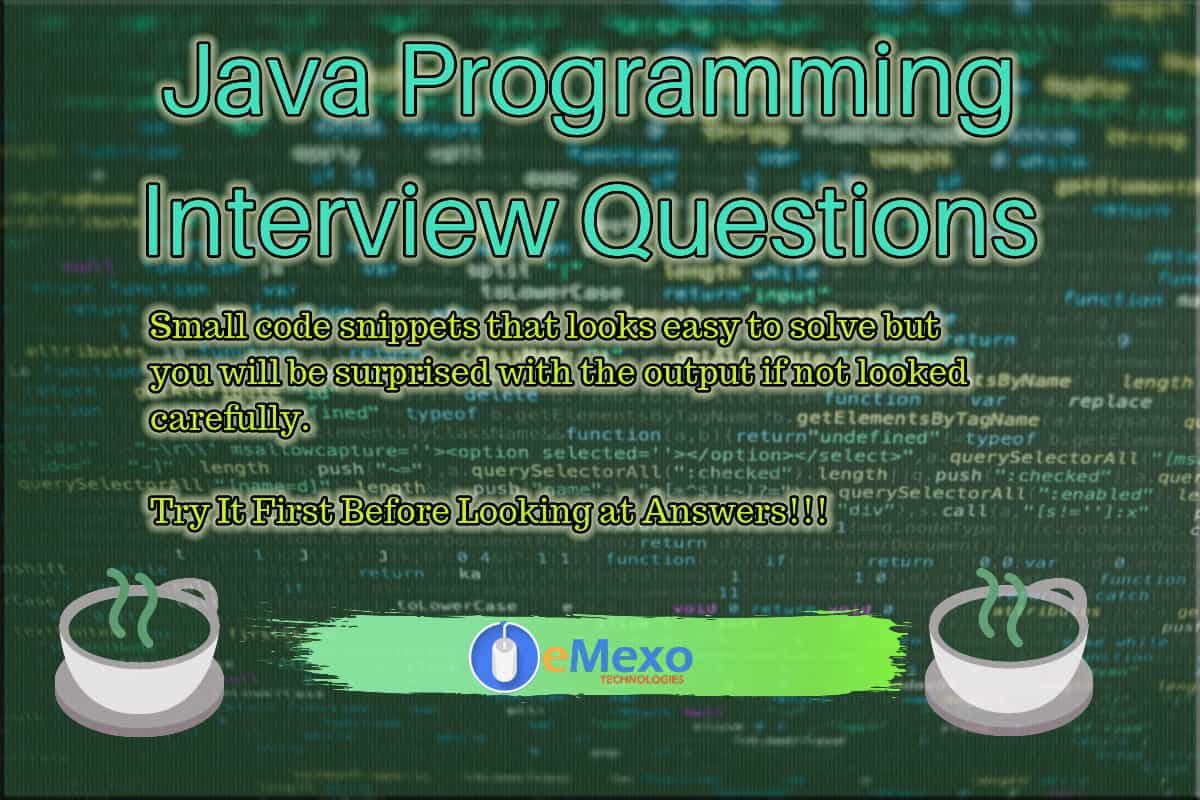
This blog is provided by eMexo Technologies with a list of basic Java coding interview questions and examples of real logic code quizzed in programming and coding interviews for freshers and experienced candidates.
1. Write the program to print the pyramid pattern of the symbol with the left alignment?
public class Pattern { |
Output:
# ## ### #### ##### |
2. Write the program to sort the array in descending order using Bubble sort?
import java.util.Arrays; public class BubbleSortDsc { public static void main(String[] args) { int[] inputArray = {34,62,6,89,3,7,23}; System.out.println(“Unsorted Array : ” + Arrays.toString(inputArray)); int[] output = sort(inputArray); System.out.println(“Sorted Array : ” + Arrays.toString(output)); } public static int[] sort(int[] inputArray) { int length = inputArray.length; for (int i = 0; i < length -1; i++) { for (int j = 0; j < length-1-i; j++) { if (inputArray[j] < inputArray[j+1]) { int tmp = inputArray[j]; inputArray[j] = inputArray[j+1]; inputArray[j+1] = tmp; } } } return inputArray; } } |
Output:
Unsorted Array : [34, 62, 6, 89, 3, 7, 23] Sorted Array : [89, 62, 34, 23, 7, 6, 3] |
3. Write the program to reverse a string with maintaining a special character position?
public class ReverseString1 { public static void main(String[] args) { String input = “Welcome to eMexo”; System.out.println(“Input String : ” + input); String output = reverse(input); System.out.println(“Reversed String: ” + output); } /** * Reverse a string with maintaining special character position. * Algorithm : * 1) Let input string be ‘inputArray[]’ and length of string be ‘length’ * 2) left = 0, right = length-1 * 3) While left is smaller than right, do following * a) If inputArray[left] is not an alphabetic character, do left++ * b) Else If inputArray[right] is not an alphabetic character, do right– * c) Else swap inputArray[left] and inputArray[right] * * @param input : Input string * @return reverse string */ public static String reverse(String input) { char[] inputArray = input.toCharArray(); // length of the array int length = inputArray.length; int left = 0; int right = length -1; while (left < right) { // check if the first character is special character if (String.valueOf(inputArray[left]).matches(“[^a-z A-Z 0-9]”)) { left++; // check if the last character is special character } else if (String.valueOf(inputArray[right]).matches(“[^a-z A-Z 0-9]”)) { right–; } else { char temp = inputArray[left]; inputArray[left] = inputArray[right]; inputArray[right] = temp; left++; right–; } } return String.valueOf(inputArray); } } |
Output:
Input String: Welcome to eMexo Reversed String: oxeMe ot emocleW |
4. How to convert Map into List?
import java.util.ArrayList; import java.util.HashMap; import java.util.List; import java.util.Map.Entry; /** *Converting HashMap into ArrayList in Java */ public class MaptoListJava { public static void main(String… args) { HashMap<String, String> personalLoanOffers = new HashMap<String, String>(); // preparing hashmap with keys and values personalLoanOffers.put(“personal loan by DBS”, “Interest rate low”); personalLoanOffers.put(“personal loan by Standard Charted”, “Interest rate low”); personalLoanOffers.put(“HSBC personal loan by DBS”, “14%”); personalLoanOffers.put(“Bankd of America Personal loan”, “11%”); System.out.println(“Size of personalLoanOffers Map: ” + personalLoanOffers.size()); //Converting HashMap keys into ArrayList List<String> keyList = new ArrayList<String>(personalLoanOffers.keySet()); System.out.println(“Size of Key list from Map: ” + keyList.size()); //Converting HashMap Values into ArrayList List<String> valueList = new ArrayList<String>(personalLoanOffers.values()); System.out.println(“Size of Value list from Map: ” + valueList.size()); List<Entry> entryList = new ArrayList<Entry>(personalLoanOffers.entrySet()); System.out.println(“Size of Entry list from Map: ” + entryList.size()); } } |
Output:
Size of personalLoanOffers Map: 4 Size of Key list from Map: 4 Size of Value list from Map: 4 Size of Entry list from Map: 4 |
5. Java Program to check if a vowel is present in the string?
public class StringContainsVowels { public static void main(String[] args) { System.out.println(stringContainsVowels(“Let us”)); // true System.out.println(stringContainsVowels(“Try”)); // false } public static boolean stringContainsVowels(String input) { return input.toLowerCase().matches(“.*[aeiou].*”); } } |
Output:
true false |
6. Java program to check if the given number is prime?
public class PrimeNumberCheck { public static void main(String[] args) { System.out.println(isPrime(17)); // true System.out.println(isPrime(777)); // false } public static boolean isPrime(int n) { if (n == 0 || n == 1) { return false; } if (n == 2) { return true; } for (int i = 2; i <= n / 2; i++) { if (n % i == 0) { return false; } } return true; } } |
Output:
true false |
7. Java program to check if the given number/string is Palindrome or not?
import java.util.*; class PalindromeExample2 { public static void main(String args[]) { String original, reverse = “”; // Objects of String class Scanner in = new Scanner(System.in); System.out.println(“Enter a string/number to check if it is a palindrome”); original = in.nextLine(); int length = original.length(); for ( int i = length – 1; i >= 0; i– ) reverse = reverse + original.charAt(i); if (original.equals(reverse)) System.out.println(“Entered string/number is a palindrome.”); else System.out.println(“Entered string/number isn’t a palindrome.”); } } |
Output:
Enter a string/number to check if it is a palindrome eMexo Technologies Entered string/number isn’t a palindrome. |
8. Write the Java program to get Fibonacci Series?
class FibonacciExample1{ public static void main(String args[]) { int n1=0,n2=1,n3,i,count=10; System.out.print(n1+” “+n2); //printing 0 and 1 for(i=2;i<count;++i) //loop starts from 2 because 0 and 1 are already printed { n3=n1+n2; System.out.print(” “+n3); n1=n2; n2=n3; } } } |
Output:
0 1 1 2 3 5 8 13 21 34 |
9. Write the Java program to find the Factorial of a number?
import java.util.; class FactorialExample { public static void main(String args[]){ int i,fact=1; Scanner a = new Scanner (System.in); System.out.print(“Enter the number to find its factorial: “); int number = a.nextInt(); //It is the number to calculate factorial for(i=1;i<=number;i++){ fact=facti; } System.out.println(“Factorial of “+number+” is: “+fact); } } |
Output:
Enter the number to find its factorial: 7 Factorial of 7 is: 5040 |
10. Write the Java program to find the Armstrong Number?
import java.util.Scanner; import java.lang.Math; public class ArmstsrongNumberExample { //function to check if the number is Armstrong or not static boolean isArmstrong(int n) { int temp, digits=0, last=0, sum=0; //assigning n into a temp variable temp=n; //loop execute until the condition becomes false while(temp>0) { temp = temp/10; digits++; } temp = n; while(temp>0) { //determines the last digit from the number last = temp % 10; //calculates the power of a number up to digit times and add the resultant to the sum variable sum += (Math.pow(last, digits)); //removes the last digit temp = temp/10; } //compares the sum with n if(n==sum) //returns if sum and n are equal return true; //returns false if sum and n are not equal else return false; } //driver code public static void main(String args[]) { int num; Scanner sc= new Scanner(System.in); System.out.print(“Enter the limit: “); //reads the limit from the user num=sc.nextInt(); System.out.println(“Armstrong Number up to “+ num + ” are: “); for(int i=0; i<=num; i++) //function calling if(isArmstrong(i)) //prints the armstrong numbers System.out.print(i+ “, “); } } |
Output:
Enter the limit: 777 Armstrong Number up to 777 are: 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 153, 370, 371, 407, |