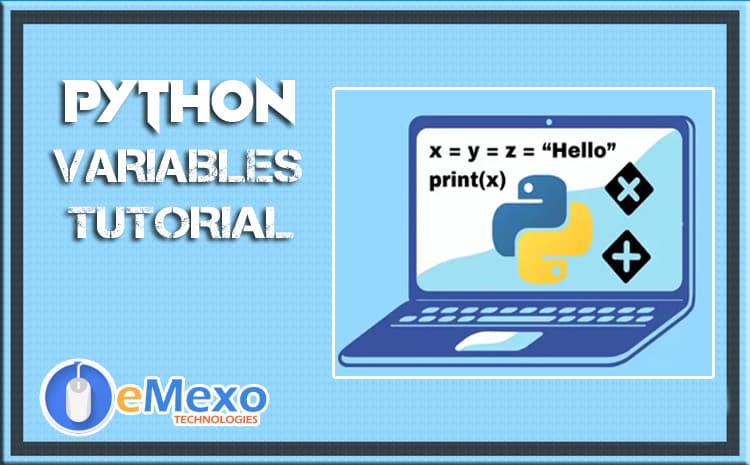
The variable is the name used to refer to the memory location. This means that you reserve memory when you create the variable. Python variables, also known as identifiers, are used to hold values. Python is an inference language and is smart enough to get the type of a variable, so you don’t need to specify the type of the variable. The value stored in the variable can be changed while the program is running.
Rules to create Python variables:
- First, Variable names must start with a letter or an underscore.
- Second, Variable names cannot start with a number.
- Third, Variable names can only contain alphanumerics and underscores (A-Z, 0-9, and _).
- Then, Variable names are case sensitive (Name, Name, and NAME are three different variables).
- Finally, Variables cannot be named using reserved words (keywords).
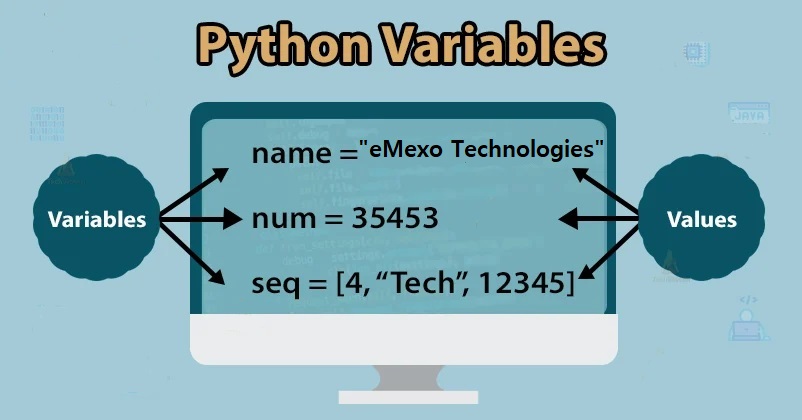
Let’s take a look at creating a simple variable.
#!/usr / bin / python
# An integer assignment
age = 45
# A floating point
salary = 1456.8
# A string
name = "John"
print(age)
print(salary)
print(name)
Output:
45
1456.8
John
Declare the Variable:
Let’s see how to declare a variable and how to print a variable.
# declaring the var
Number = 100
# display
print( Number)
Output:
100
Re-declare the Variable:
After declaring the variable, you can redeclare the Python variable.
For Example:
# declaring the var
Number = 100
# display
print("Before declare: ", Number)
# re-declare the var
Number = 120.3
print("After re-declare:", Number)
Output:
Before declare: 100
After re-declare: 120.3
Multiple Assignment
In Python, you can assign values to multiple variables in a single statement. This is also known as multiple assignments.
You can apply multiple assignments in two ways: assigning a single value to multiple variables or assigning multiple values to multiple variables. Consider the following example.
Assigning a single value to multiple variables
For Example:
x=y=z=70
print(x)
print(y)
print(z)
Output
70
70
70
Assigning multiple values to multiple variables:
For Example:
a,b,c=5,17,eMexo
print a
print b
print c
Output
5
17
eMexo
The values are assigned in the order in which the variables are displayed.
Can we use the same name for different types?
If you use the same name, the variable will start referencing a new value and type.
For Example:
#!/usr / bin / python
a = 10
a = "eMexo Technologies"
print(a)
Output:
eMexo Technologies
How does the + operator work with variables?
For Example:
#!/usr / bin / python
a = 17
b = 10
print(a+b)
a = "eMexo"
b = "Technologies"
print(a+b)
Output:
27
eMexoTechnologies
Can we use + for different types also?
No, using different types would produce an error.
For Example:
#!/usr / bin / python
a = 10
b = "eMexo"
print(a+b)
Output:
TypeError: unsupported operand type(s) for +: 'int' and 'str'
Python Variable Types
There are two types of variables in Python: local variables and global variables. Let’s understand the following variables.
Local Variable
Local variables are variables that are declared within a function and have scope within the function. Let’s understand the following example.
For Example:
# Declaring a function
def add():
# Defining local variables. They has scope only within a function
a = 20
b = 30
c = a + b
print("The sum is:", c)
# Calling a function
add()
Output:
The sum is: 50
The explanation for the code:
In the code above, I declared a function called add() and assigned some variables inside the function. These variables are called local variables and are only valid within the function. If you try to use them outside the function, you will get the following error:
add()
# Accessing local variable outside the function
print(a)
Output:
The sum is: 50
print(a)
NameError: name 'a' is not defined
We tried to use a local variable outside their scope; it threw the NameError.
Global Variables
Global variables can be used throughout the program, and its scope is in the entire program. We can use global variables inside or outside the function. Variables declared outside the function are global variables by default. Python provides global keywords for using global variables within functions. If you do not use the global keyword, the function treats it as a local variable. Let’s understand the following example.
For Example:
# Declare a variable and initialize it
x = 101
# Global variable in function
def mainFunction():
# printing a global variable
global x
print(x)
# modifying a global variable
x = 'Welcome To eMexo Technologies'
print(x)
mainFunction()
print(x)
Output:
101
Welcome To eMexo Technologies
Welcome To eMexo Technologies
The explanation for the code:
The code above declares the global variable x and assigns it a value. Next, I defined the function and accessed the variables declared using the global keywords in the function. You can now change that value. Then I assigned the variable x a new string value.
Now the function is called and we proceed to the print of x. Output the newly assigned value of x.
Delete a variable
You can delete a variable using the del keyword. The syntax is as follows:
Syntax :
del <variable_name>
The following example creates the variable x and assigns it a value. If you delete the variable x and output it, you will get the error “Variable x is not defined“. The variable x will no longer be used.
For Example:
# Assigning a value to x
x = 7
print(x)
# deleting a variable.
del x
print(x)
Output:
7
Traceback (most recent call last):
File "C:/Users/eMexo/PycharmProjects/Hello/multiprocessing.py", line 6, in
print(x)
NameError: name 'x' is not defined
Maximum Possible Value of an Integer in Python
Unlike other programming languages, Python does not have long int or float data types. Treat all integer values as int data types. The problem arises here. What is the maximum value a variable can hold in Python? Consider the following example.
For Example:
# A Python program to display that we can store
# large numbers in Python
a = 10000000000000000000000000000000000000000000
a = a + 1
print(type(a))
print (a)
Output:
<class 'int'>
10000000000000000000000000000000000000000001
As you can see in the example above, we assigned a large integer value to the variable x and checked its type. Class long int was not printed instead of that int is printed. Therefore, there is no limit to the number of bits and it can be extended to the memory limit. Python has no special data type for storing larger numbers.