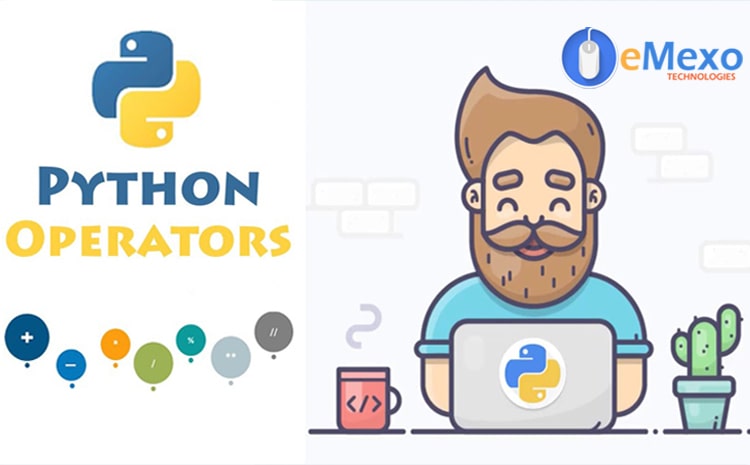
An operator can be defined as a symbol responsible for a particular operation between two operands. The following are some symbols ( -+, *, /) used as Python operators. Each symbol or operator has its own type of operation. The value at which an operator performs each operation is called an operand. This article describes different types of Python operators.
Types of Python Operators
Python language supports the following types of operators:
- Arithmetic operators
- Comparison operators
- Assignment Operators
- Logical Operators
- Bitwise Operators
- Membership Operators
- Identity Operators
Arithmetic Operators
Arithmetic operators are used to performing mathematical operations such as addition, subtraction, multiplication, and division.
Operator | Description | For Example |
---|---|---|
+ (Addition) | It is used to add two operands. | If a = 20, b = 10 => a+b = 30 |
– (Subtraction) | It is used to subtract the second operand from the first operand. If the first operand is less than the second operand, the result will be negative. | If a = 20, b = 10 => a – b = 10 |
/ (divide) | Divides the first operand by the second operand and then returns the quotient. | If a = 20, b = 10 => a/b = 2.0 |
* (Multiplication) | It is used to multiply one operand with the other. | If a = 20, b = 10 => a * b = 200 |
% (reminder) | Returns memory after dividing the first operand by the second operand. | If a = 20, b = 10 => a%b = 0 |
** (Exponent) | This is the exponential operator displayed to calculate the power of the second operand of the first operand. | If a=4, b=20 =>a /b => 204 |
// (Floor division) | It specifies the minimum quotient resulting from the division of two operands. | If a=30, b=20 =>a // b => 1 |
Consider the following example Program for Arithmetic Operator:
# Examples of Arithmetic Operator
a = 9
b = 4
# Addition of numbers
add = a + b
# Subtraction of numbers
sub = a - b
# Multiplication of number
mul = a * b
# Division(float) of number
div1 = a / b
# Division(floor) of number
div2 = a // b
# Modulo of both number
mod = a % b
# Power
p = a ** b
# print results
print(add)
print(sub)
print(mul)
print(div1)
print(div2)
print(mod)
print(p)
Output:
13
5
36
2.25
2
1
6561
Comparison Operators
Python Comparison operators are also called Relational operators. They compare the values on both sides of the operator and infer the relationships between the values. After comparison, returns a Boolean value, Such as either true or false.
The following table contains various types of comparison operators, their descriptions, and corresponding examples.
Operator | Description | For Example |
---|---|---|
== (Equal to) | The condition is true if the values of the two operands are equal. | If I = 20, J = 20 (I == J) is True |
!= (Not Equal to) | If the values of the two operands are not equal, the condition is true. | If I = 20, J = 20 (I != J) is False |
<> (Not equal to (similar to !=)) | If the values of the two operands are not equal, the condition is true. | If I=40, J = 20 (I <> J) is True. |
> (Greater than) | The condition is true if the value of the left operand is greater than the value of the right operand. | If I= 40, J = 20 (I > J) is True |
< (Less than) | The condition is true if the value of the left operand is less than the value of the right operand. | If I = 40, J = 20 (I < J) is False |
>= (Greater than or equal to) | If the value of the left operand is greater than or equal to the value of the right operand, true is returned. | If I = 40, J = 20 (I >= J) is True |
<= (Less than or equal to) | If the value of the left operand is less than or equal to the value of the right operand, true is returned. | If I = 40, J = 20 (I <= J) is False |
Consider the following example program for Comparison Operators in Python
# Examples of Relational Operators
a = 13
b = 33
# a > b is False
print(a > b)
# a < b is True
print(a < b)
# a == b is False
print(a == b)
# a != b is True
print(a != b)
# a >= b is False
print(a >= b)
# a <= b is True
print(a <= b)
Output:
False
True
False
True
False
True
Python Assignment Operators
The assignment operator is used to assign a value to a Python variable. The assignment may be made directly, or the operator may first perform some mathematical operation and then assign the value to the operand.
The following table contains all types of assignment operators, their descriptions, and corresponding examples.
Operator | Description | For Example |
---|---|---|
= (Assignment) | It assigns the value of the right expression to the left operand. | If a = 10, b = 20 => a = b => a = 20 |
+= (Add AND assign) | Increases the value of the left operand by the value of the right operand and reassigns the changed value to the left operand. | If a = 10, b = 20 => a+ = b will be equal to a = a+ b => a = 30 |
-= (Subtract AND assign) | Decrease the value of the left operand by the value of the right operand and reassign the changed value to the left operand. | If a = 20, b = 10 => a- = b will be equal to a = a- b => a = 10 |
*= (Multiply AND assign) | Multiplies the value of the left operand by the value of the right operand, then returns the changed value to the left operand. | If a = 10, b = 20 => a* = b will be equal to a = a* b => a = 200 |
/= (Divide AND assign) | Divides the value of the left operand by the value of the right operand and returns the result in the left operand. | If a = 20, b = 10 => a / = b will be equal to a = a / b => a = 2 |
%= (Modulus AND assign) | Modulus the value of the left operand by the value of the right operand and returns the result in the left operand. | If a = 20, b = 10 => a % = b will be equal to a = a % b => a = 0 |
**= (Exponent AND assign) | Use the operands to calculate the value of the exponent (power) and assign the value to the left operand. | If a = 4, b =2 =>a**=b will be equal to a = a**b => a = 16 |
//= (Floor division AND assign) | Divide the left operand by the right operand and assign the value (ground) to the left operand | if a = 4, b = 3 => a//=b will be equal to a= a//b => a = 1 |
Consider the following example program for Assignment Operators in Python
#!/usr/bin/python
a = 21
b = 10
c = 0
c = a + b
print "Line 1 - Value of c is ", c
c += a
print "Line 2 - Value of c is ", c
c *= a
print "Line 3 - Value of c is ", c
c /= a
print "Line 4 - Value of c is ", c
c = 2
c %= a
print "Line 5 - Value of c is ", c
c **= a
print "Line 6 - Value of c is ", c
c //= a
print "Line 7 - Value of c is ", c
Output:
Line 1 - Value of c is 31
Line 2 - Value of c is 52
Line 3 - Value of c is 1092
Line 4 - Value of c is 52
Line 5 - Value of c is 2
Line 6 - Value of c is 2097152
Line 7 - Value of c is 99864
Logical Operators in Python
Logical operators are primarily used in conditional statements. There are three types of logical operators: AND, OR, and NOT.
The following table contains all logical operators, their descriptions, and examples of each.
Operator | Description | Example |
---|---|---|
and | If both the operands are true then condition becomes true. | If a and b are the two expressions, a → true, b → true => a and b → true |
or | If any of the two operands are non-zero then condition becomes true. | If a and b are the two expressions, a → true, b → false => a or b → true |
not | Used to reverse the logical state of its operand. | If a and b are the two expressions, Not(a and b) => false. |
Consider the following example program for Logical Operators in Python
# Examples of Logical Operator
a = True
b = False
# Print a and b is False
print(a and b)
# Print a or b is True
print(a or b)
# Print not a is False
print(not a)
Output:
False
True
False
Python Bitwise Operators
Bitwise operators perform bitwise operations on the values of two operands. These are used to operate on binary numbers.
Operator | Description | For Example (a = 0011 1100 b = 0000 1101) |
---|---|---|
& (Binary AND) | If both bits in the same place on the two operands are 1, 1 is copied to the result. Otherwise, 0 is copied. | (a & b) (means 0000 1100) |
| (Binary OR) | If both bits are zero, the resulting bit will be zero. Otherwise, the resulting bit is 1. | (a | b) = 61 (means 0011 1101) |
^ (Binary XOR) | If both bits are different, the resulting bit will be 1. Otherwise, the resulting bit is 0. | (a ^ b) = 49 (means 0011 0001) |
~ (Binary Ones Complement) | Compute the negation of each bit of the operand. Therefore If the bit is 0, the resulting bit will be 1 and vice versa. | (~a ) = -61 (means 1100 0011 in 2’s complement form due to a signed binary number |
<< (Binary Left Shift) | The value of the left operand is shifted to the left by the number of bits present in the right operand. | a << 2 = 240 (means 1111 0000) |
>> (Binary Right Shift) | The left operand is shifted to the right by the number of bits present in the right operand. | a >> 2 = 15 (means 0000 1111) |
Consider the following example program for Bitwise Operators in Python
# Examples of Bitwise operators
a = 10
b = 4
# Print bitwise AND operation
print(a & b)
# Print bitwise OR operation
print(a | b)
# Print bitwise NOT operation
print(~a)
# print bitwise XOR operation
print(a ^ b)
# print bitwise right shift operation
print(a >> 2)
# print bitwise left shift operation
print(a << 2)
Output:
0
14
-11
14
2
40
Python Membership Operators
The membership operator is used to test whether a value is available in a sequence. It can be any sequence like (Python string, Python list, Python set, Python taple, Python dictionary). There are two types of membership operators, in and not in.
The following table describes both membership operators and examples of each.
Operator | Description | Example |
---|---|---|
in | If the first operand is found in the second operand (list, tuple, or dictionary), it evaluates to true. | x in y, here in results in a 1 if x is a member of sequence y |
not in | If the first operand is not found in the second operand (list, tuple, or dictionary), it evaluates to true. | x not in y, here not in results in a 1 if x is not a member of sequence y |
Consider the following example program for Membership Operator
# Python program to illustrate
# not 'in' operator
x = 24
y = 20
list = [10, 20, 30, 40, 50]
if (x not in list):
print("x is NOT present in given list")
else:
print("x is present in given list")
if (y in list):
print("y is present in given list")
else:
print("y is NOT present in given list")
Output:
x is NOT present in given list
y is present in given list
Python Identity Operators
The Identity operator is used to compare the memory addresses of two different objects. The two types of Identity operators in Python are is and not.
The following table contains a description of these two operators and an example of each.
Operator | Description | Example |
---|---|---|
is | Evaluates to true if the references on both sides point to the same object. | x is y, here is results in 1 if id(x) equals id(y) |
is not | Evaluates to true if the references on both sides do not point to the same object. | x is not y, here is not results in 1 if id(x) is not equal to id(y) |
Consider the following example program for Identity Operator
a = 10
b = 20
c = a
print(a is not b)
print(a is c)
Output:
True
True
Python Operator Precedence
Some expressions have multiple operators. To evaluate such expressions, there is a precedence rule called the Python operator precedence. Controls the order in which these operations are performed.
The following table shows the operator precedence in Python in descending order.
Operator | Description |
---|---|
** | The exponential operator takes precedence over all other operators used in expressions. |
~ + – | The negation, unary plus, and minus. |
* / % // | The multiplication, divide, modules, reminder, and floor division. |
+ – | Binary plus, and Binary minus |
>> << | Left shift. and right shift |
& | Binary and |
^ | | Binary xor, and or |
<= < > >= | Comparison operators (less than, less than equal to, greater than, greater then equal to) |
<> == != | Equality operators |
= %= /= //= -= += *= **= | Assignment operators |
is is not | Identity operators |
in not in | Membership operators |
not or and | Logical operators |
Consider the following example Program:
#!/usr/bin/python
a = 20
b = 10
c = 15
d = 5
e = 0
e = (a + b) * c / d #( 30 * 15 ) / 5
print "Value of (a + b) * c / d is ", e
e = ((a + b) * c) / d # (30 * 15 ) / 5
print "Value of ((a + b) * c) / d is ", e
e = (a + b) * (c / d); # (30) * (15/5)
print "Value of (a + b) * (c / d) is ", e
e = a + (b * c) / d; # 20 + (150/5)
print "Value of a + (b * c) / d is ", e
Output:
Value of (a + b) * c / d is 90
Value of ((a + b) * c) / d is 90
Value of (a + b) * (c / d) is 90
Value of a + (b * c) / d is 50