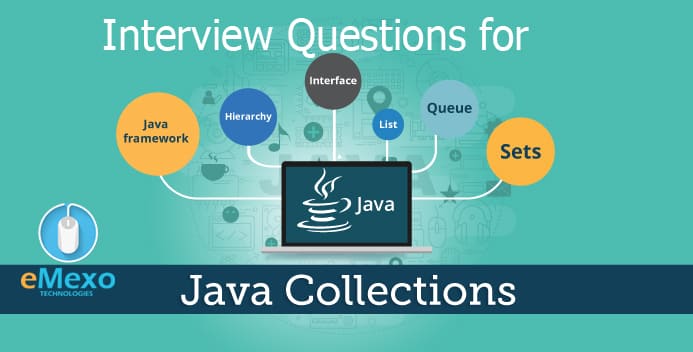
This is an article about top 10 JAVA Collections Interview Questions for both fresh and experienced candidates to get their dream job.
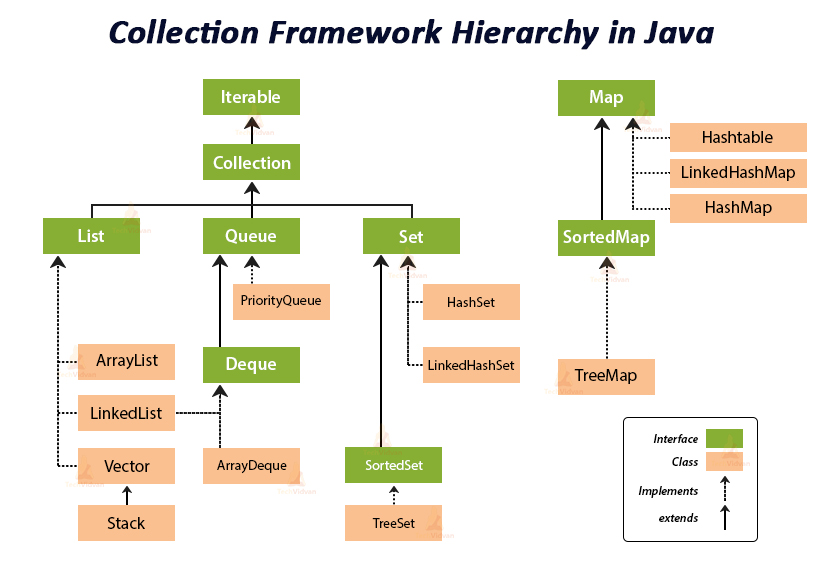
Enroll Java training in Electronic City Bangalore with eMexo Technologies today, get Java certification, and start your career at your dream company.
1. What is the Collection framework in Java?
The collection framework is a combination of classes and interfaces used to store and manipulate data in the form of objects. To do this, we provide various classes such as ArrayList, Vector, Stack, HashSet, and interfaces such as List, Queue, and Set.
2. What is the framework in Java?
A framework is a general, pre-built architecture that contains a set of classes and interfaces.
3. What do you mean by Collections Class?
java.util.Collections is a class that consists of static methods for manipulating collections. But it contains polymorphic algorithms for manipulating collection “wrappers”. This class contains methods for algorithms such as binary sorting, searching, and shuffling.
4. Define BlockingQueue?
BlockingQueue is an interface used in Java that allows you to extend a queue. It provides concurrency for various queue operations such as fetch, inserts, and delete. The queue is waiting not to be empty when fetching an item. But it does not include null elements in the BlockingQueue. Therefore the implementation of this queue is thread-safe. Finally, the syntax for the BlockingQueue is:
public interface BlockingQueue<E> extends Queue <E> |
5. Explain the override equals() method?
The equals method is used to check the similarity between two objects. But if the programmer validates the object based on its properties, it should be overridden.
6. What is the advantage of the generic collection?
There are three main advantages of using the generic collection.
- If we use the generic class, we don’t need typecasting.
- It is type-safe and checked at compile time.
- Generic confirms the stability of the code by making it bug detectable at compile time.
7. How to reverse ArrayList?
You can use the reverse () method of the Collections class to reverse the ArrayList. Consider the following example.
import java.util.ArrayList; import java.util.Collection; import java.util.Collections; import java.util.Iterator; import java.util.List; public class ReverseArrayList { public static void main(String[] args) { List list = new ArrayList<>(); list.add(10); list.add(50); list.add(30); Iterator i = list.iterator(); System.out.println(“printing the list….”); while(i.hasNext()) { System.out.println(i.next()); } Iterator i2 = list.iterator(); Collections.reverse(list); System.out.println(“printing list in reverse order….”); while(i2.hasNext()) { System.out.println(i2.next()); } } } |
Output: printing the list… 10 50 30 printing list in reverse order… 30 50 10 |
8. What is ConcurrentHashMap?
- The Java 1.5 concurrency package introduces concurrentHashMap as an alternative to Hashtable.
- There were only two options for concurrent, multi-threaded Java programs using a Map implementation prior to Java 1.5: Hashtable or synchronizedMap since HashMap is not thread-safe.
- The advantage of ConcurrentHashMap is that you can safely use it in concurrent multi-threaded environments, as well as get better performance over Hashtable and SynchronizedMap.
- In comparison to Hashtable and Synchronized Map, ConcurrentHashMap performs better because it only locks part of the Map, instead of the whole one, as it would with a Hashtable.
- As well as concurring reads and writes, CHM maintains integrity by synchronizing writes.
- Due to a lack of synchronization, operations like put(), remove(), putAll(), and clear() might not reflect the current state of the map.
- In the case of putAll() or clear(), which operates on the whole Map, concurrent read may reflect the insertion and removal of only some entries.
- It is also important to remember that iteration over CHM is weekly consistent and iterators returned by keySet of ConcurrentHashMap may not reflect recent changes.
- Iterator of ConcurrentHashMap’s keySet area is also fail-safe and doesn’t throw ConcurrentModificationExceptoin.
//Initialize ConcurrentHashMap instance ConcurrentHashMap<String, Integer> m = new ConcurrentHashMap<String, Integer>(); //Print all values stored in ConcurrentHashMap instance for each (Entry<String, Integer> e : m.entrySet()) { system.out.println(e.getKey()+”=”+e.getValue()); } |
The above code is reasonably valid in a multi-threaded environment in your application.
The reason, I am saying “reasonably valid” is that the above code yet provides thread safety, still it can decrease the performance of an application. And ConcurrentHashMap was introduced to improve the performance while ensuring thread safety, right?
So, what is that we are missing here??
To understand that we need to understand the internal working of ConcurrentHashMap class. Finally, the best way to start is to look at the constructor arguments.
In addition, A fully parameterized constructor of ConcurrentHashMap takes 3 parameters, initialCapacity, loadFactor, and concurrency level.
- initialCapacity
- loadFactor
- concurrencyLevel
The first two are fairly simple as their name implies but the last one is the tricky part. This denotes the number of shards. It is used to divide the ConcurrentHashMap internally into this number of partitions and the equal number of threads are created to maintain thread safety maintained at the shard level.
The default value of “concurrencyLevel” is 16. It means 16 shards whenever we create an instance of ConcurrentHashMap using the default constructor, before even adding the first key-value pair.
It also means the creation of instances for various inner classes like ConcurrentHashMap$Segment, ConcurrentHashMap$HashEntry[] and ReentrantLock$NonfairSync.
In any case in normal applications, a single shard is able to handle multiple threads with a reasonable count of key-value pairs. And performance will be also optimal. Similarly having multiple shards just makes things complex internally and introduces a lot of unnecessary objects for garbage collection, and all this for no performance improvement.
The extra objects created per concurrent hashmap using the default constructor are normally in the ratio of 1 to 50 i.e. for 100 such instances of ConcurrentHashMap, there will be 5000 extra objects created.
Similarly based on the above, I will suggest using the constructor parameters wisely to reduce the number of unnecessary objects and improve the performance.
A good approach can be having initialization like this:
ConcurrentHashMap<String, Integer> instance = new ConcurrentHashMap<String, Integer>(16, 0.9f, 1);
An initial capacity of 16 ensures a reasonably good number of elements before resizing happens. Also, a load factor of 0.9 ensures a dense packaging inside ConcurrentHashMap which will optimize memory use. Finally concurrencyLevel set to 1 will ensure that only one shard is created and maintained.
Please note that if you are working on a very high concurrent application with a very high frequency of updates in ConcurrentHashMap, you should consider increasing the concurrencyLevel more than 1, but again it should be a well-calculated number to get the best results.
Summary
- At first, ConcurrentHashMap allows concurrent read and thread-safe update operation.
- During the update operation, ConcurrentHashMap only locks a portion of the Map instead of the whole Map.
- The concurrent update is achieved by internally dividing Map into the small portion which is defined by concurrency level.
- Choose concurrency level carefully as a significantly higher number can be a waste of time and space and the lower number may introduce thread contention in case of writers over number concurrency level.
- In short, All operations of ConcurrentHashMap are thread-safe.
- Since ConcurrentHashMap implementation doesn’t lock the whole Map, there is a chance of reading overlapping with update operations like put() and remove(). In that case, the result returned by get() method will reflect the most recently completed operation from their start.
- Thus the Iterator returned by ConcurrentHashMap is weekly consistent, fail-safe, and never throws ConcurrentModificationException. In Java.
- However, ConcurrentHashMap doesn’t allow null as a key or value.
- You can use ConcurrentHashMap in place of Hashtable but with caution as CHM doesn’t lock the whole Map.
- During putAll() and clear() operations, the concurrent read may only reflect the insertion or deletion of some entries.
9. What are the benefits of the Collection Framework in Java?
Thus the advantages of the Java collection framework are:
- Since Java Collection Framework provides highly efficient and effective data structures that improve the accuracy and speed of your programs.
- In addition Developed using the Java Collection Framework, this program is easy to maintain.
- In short, Developers can mix classes with other types, which improves code reusability.
- Finally, the Java collection framework gives programmers the freedom to change the primitive collection type.
10. Java Concurrent Collection – CopyOnWriteArrayList Examples?
Basically, a CopyOnWriteArrayList is similar to an ArrayList, with some additional and more advanced thread-safe features.
You know, ArrayList is not thread-safe so it’s not safe to use in multi-threaded applications. Finally We can achieve a thread-safe feature for an ArrayList by using a synchronized wrapper like this:
List<String> unsafeList = new ArrayList<>(); List<String> safeList = Collections.synchronizedList(unsafeList); safeList.add(“Boom”); // safe to use with multiple threads |