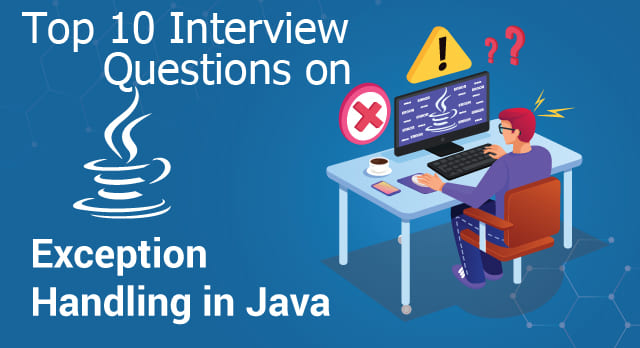
This is an article on the Top 10 Java Exception Handling Interview Questions for both fresh and experienced candidates to get you started with your dream job.
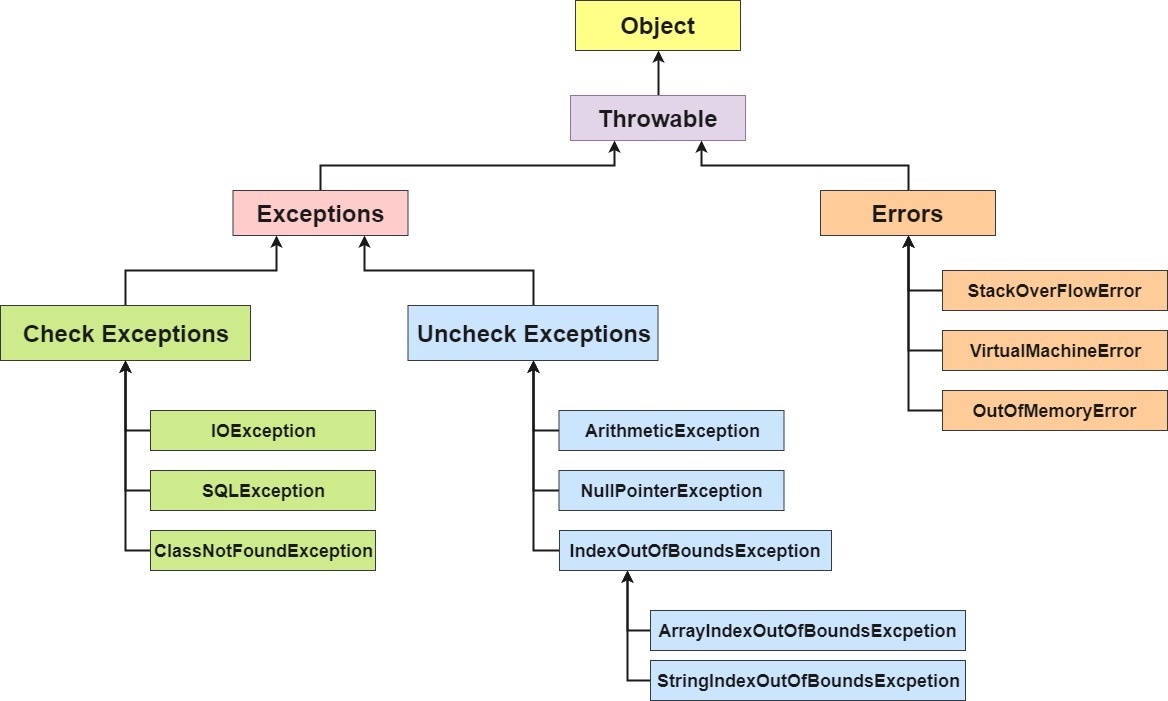
Finally, Start your career at your dream company with eMexo Technologies now, enroll in Java training in Electronic City Bangalore, get Java certification, and start your career at your dream company.
1. How to create a custom Exception?
- At first, We can create our own exceptions by extending the ‘Exception’ class
- Secondly, We can create checked or unchecked custom exception
- While creating a custom exception, prefer to create an unchecked, Runtime exception than a checked exception, especially if you know that client is not going to take any reactive action other than logging.
- Apart from providing a default no-argument constructor on your custom Exception class, consider providing at least two more constructors, one which should accept a failure message and the other which can accept another Throwable as the cause
public class MyOwnException { public static void main(String[] a){ try{ MyOwnException.myTest(null); } catch(MyAppException mae){ System.out.println(“Inside catch block: “+mae.getMessage()); } } static void myTest(String str) throws MyAppException{ if(str == null){ throw new MyAppException(“String val is null”); } } } class MyAppException extends Exception { private String message = null; public MyAppException() { super(); } public MyAppException(String message) { super(message); this.message = message; } public MyAppException(Throwable cause) { super(cause); } @Override public String toString() { return message; } @Override public String getMessage() { return message; } } |
2. What’s the difference between throws and throw keywords?
Definition:
The throw is a statement and is used in a method to explicitly throw an exception.
The throws keyword is used by a method to specify which exceptions can be thrown from the method.
Syntax:
If we see syntax wise then throw is followed by an instance of Exception class and throws is followed by exception class names.
For Example:
throw new ArithmeticException(“Arithmetic Exception”); |
throws ArithmeticException; |
Type of exception:
You can use the Throw keyword to propagate only unchecked exceptions. Therefore Checked exceptions cannot be propagated by throw.
On the other hand, both checked and unchecked exceptions can be declared using the throws keyword. For propagation, checked exceptions must use the specific exception class name after the throws keyword.
Declaration:
To use the throw keyword, you need to know that the throw keyword is used in the method.
On the other hand, the throws keyword is used in the method signature.
Example Program:
Throw statement example given below:
public class ThrowExample { public static void checkPassEligibility(float totalMarks) { if(totalMarks > 33) { System.out.println(“Student passed”); } else { throw new ArithmeticException(“Student failed”); } } public static void main(String[] args) { ThrowExample te = new ThrowExample(); System.out.print(“Hi! Please check your score”); te.checkPassEligibility(31); System.out.println(“Thank you!”); } } |
Output : Hi! Please check your score Exception in thread “main” java.lang.ArithmeticException: Student failed at ThrowExample.checkPassEligibility(ThrowExample.java:7) at ThrowExample.main(ThrowExample.java:13) |
Throws keyword example is given below :
public class ThrowsExample { public int division(int numerator,int denominator) throws ArithmeticException { int result = numerator/denominator; return result; } public static void main(String[] args) { ThrowsExample te=new ThrowsExample(); System.out.print(“Division of numerator and denominator : “); te.division(50,0); } } |
Output : Division of numerator and denominator : Exception in thread “main” java.lang.ArithmeticException: / by zero at ThrowsExample.division(ThrowsExample.java:4) at ThrowsExample.main(ThrowsExample.java:11) |
3. What’s the difference between checked and unchecked exceptions?
- Checked exceptions are subclasses of the Exception class, and unchecked exceptions are run-time exceptions.
- For checked exceptions, the Java virtual machine must catch or handle the exception, but for unchecked exceptions, the Java virtual machine does not need to catch or handle the exception.
- Checked exceptions are checked at program run time, and unchecked exceptions are checked at program compile time.
- Both checked and unchecked exceptions can be created manually.
- Both checked and unchecked exceptions can be handled with a try, catch, and final. The unchecked exceptions are primarily programming errors.
- Unchecked exceptions can be ignored programmatically, but unchecked exceptions cannot be ignored programmatically.
4. What’s the use of finally block?
Java finally blocks are used to contain important code such as cleanup code. For example, Close the file or close the connection. The finally block runs regardless of whether an exception has occurred and whether the exception has been processed. Finally, contains all the important statements, whether or not an exception has occurred.
Case 1: When an exception does not occur
Let’s look at the following example. The Java program does not throw an exception and the finally block is executed after the try block.
class TestFinallyBlock { public static void main(String args[]){ try{ //below code do not throw any exception int data=25/5; System.out.println(data); } //catch won’t be executed catch(NullPointerException e){ System.out.println(e); } //executed regardless of exception occurred or not finally { System.out.println(“finally block is always executed”); } System.out.println(“rest of phe code…”); } } |
Output:

Case 2: When an exception occurs but is not handled by the catch block
Let’s see the following example. Here, the code throws an exception however the catch block cannot handle it. Despite this, the finally block is executed after the try block and then the program terminates abnormally.
public class TestFinallyBlock1{ public static void main(String args[]){ try { System.out.println(“Inside the try block”); //below code throws divide by zero exception int data=25/0; System.out.println(data); } //cannot handle Arithmetic type exception //can only accept Null Pointer type exception catch(NullPointerException e){ System.out.println(e); } //executes regardless of exception occurred or not finally { System.out.println(“finally block is always executed”); } System.out.println(“rest of the code…”); } } |
Output:

Case 3: When an exception occurs and is handled by the catch block
Example:
Let’s see the following example where the Java code throws an exception and the catch block handles the exception. Later the finally block is executed after the try-catch block. Further, the rest of the code is also executed normally.
public class TestFinallyBlock2{ public static void main(String args[]){ try { System.out.println(“Inside try block”); //below code throws divide by zero exception int data=25/0; System.out.println(data); } //handles the Arithmetic Exception / Divide by zero exception catch(ArithmeticException e){ System.out.println(“Exception handled”); System.out.println(e); } //executes regardless of exception occurred or not finally { System.out.println(“finally block is always executed”); } System.out.println(“rest of the code…”); } } |
Output:
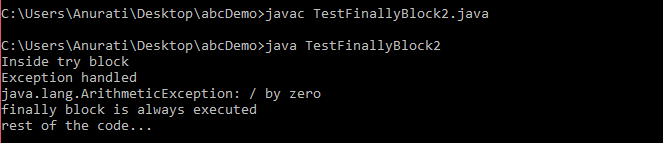
4. What’s the use of finally block in Java?
- At first, Typically, “finally” blocks or clauses are used to free resources, clean up code, connect DBs, close IO streams, and so on.
- Secondly, Java finally block used to prevent resource leaks. When you close a file or restore a resource, the code is placed in a finally block so that the resource is always restored.
- At last, The finally clause is used to terminate a thread.
5. How to catch the multiple exceptions in java?
A try block can be followed by one or more catch blocks. Each catch block must contain a different exception handler. Therefore, if you need to perform different tasks when different exceptions occur, use the Java multi-catch block.
Important Points to Remember
- Only one exception can occur at a time, and only one catch block can be executed at a time.
- All catch blocks should be sorted from the most specific to the most common. Therefore the catch of ArithmeticException must precede the catch of Exception.
Flowchart of Multi-catch Block
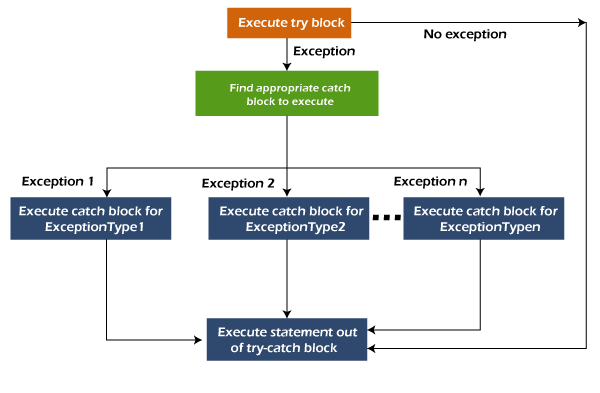
For Example:
Let’s see a simple example of java multi-catch block.
MultipleCatchBlock1.java
public class MultipleCatchBlock1 { public static void main(String[] args) { try{ int a[]=new int[5]; a[5]=30/0; } catch(ArithmeticException e) { System.out.println(“Arithmetic Exception occurs”); } catch(ArrayIndexOutOfBoundsException e) { System.out.println(“ArrayIndexOutOfBounds Exception occurs”); } catch(Exception e) { System.out.println(“Parent Exception occurs”); } System.out.println(“rest of the code”); } } |
Output:
Arithmetic Exception occurs rest of the code |
6. Can we have multiple try blocks with only one catch block in java?
A Catch block cannot have multiple Try blocks. Each try block must be followed by a catch or finally. Still, trying to create a single catch block for multiple try blocks will generate a compile-time error.
For Example, Let us compile the following Java program that tries to employ a single catch block for multiple try blocks.
class ExceptionExample { public static void main(String args[]) { int a,b; try { a=Integer.parseInt(args[0]); b=Integer.parseInt(args[1]); } try { int c=a/b; System.out.println(c); } catch(Exception ex) { System.out.println(“Please pass the args while running the program”); } } } |
Compile-time exception
ExceptionExample.java:4: error: ‘try’ without ‘catch’, ‘finally’ or resource declarations try { ^ 1 error |
7. How to avoid null pointer exception in Java?
A Null Pointer Exception is a type of run-time exception that is thrown when a Java program attempts to use an object reference that contains a null value. The following scenarios can throw a null pointer exception.

1. The method is invoked using a null object
When you call a method on a null object, the program throws a NullPointerException. Consider the following example.
|
Output

2. Passing a null object as an argument
You may get a null pointer exception if you do not check the null value in the method argument. Consider the following example.
public class Main { |
Output

3. Getting the length of a null array
Attempting to calculate the length of a null array also throws a java.lang.NullPointerException. Consider the following example.
public class Main { |
Output

The above program declares an array and assigns it null therefore No data. Using the length property on this null array throws a NullPointerException.
4. Synchronization on a null object
If you try to synchronize a method or block concurrent access, you need to ensure that the object reference used for synchronization is null. Consider the following example.
public class Main { |
Output

5. Tries to operate on the array object which is null
However, if you try to perform an operation on a null array, a null pointer exception will be thrown. Consider the following example.
public class DummyClass { int arr[] = null; public static void main(String[] args) { // TODO Auto-generated method stub DummyClass dummy = new DummyClass(); System.out.println(dummy.arr.length); } } |
Output:
Exception in thread “main” java.lang.NullPointerException: Cannot read the array length because “dummy.arr” is null at DummyClass.main(DummyClass.java:7) |
8. Why use custom exceptions in java?
Java exceptions cover almost all common types of exceptions that can occur in programming. However, you may need to create a custom exception. Here are some of the reasons for using
custom exceptions:
- To catch a subset of existing Java exceptions and handle them specifically.
- Business Logic Exceptions: These are business logic and workflow-related exceptions. It is helpful for application users or developers to understand the exact problem.
To create a custom exception, you need to extend the Exception class that belongs to the java.lang package.
Consider the following example. But in this example, we are creating a custom exception called WrongFileNameException.
public class WrongFileNameException extends Exception { public WrongFileNameException(String errorMessage) { super(errorMessage); } } |
Note: You need to create a constructor that uses the string as an error message. This is called the parent class’s constructor. |
For Example:
In addition, let’s look at a simple example of a custom Java exception. In the following code, the InvalidAgeException constructor accepts a string as an argument. Also, this string is passed to the constructor of the superclass Exception using the super () method. Also, the constructor of the Exception class can be called without parameters, and calling the super () method is not mandatory.
TestCustomException1.java
// class representing custom exception class InvalidAgeException extends Exception { public InvalidAgeException (String str) { // calling the constructor of parent Exception super(str); } } // class that uses custom exception InvalidAgeException public class TestCustomException1 { // method to check the age static void validate (int age) throws InvalidAgeException{ if(age < 18){ // throw an object of user defined exception throw new InvalidAgeException(“age is not valid to vote”); } else { System.out.println(“welcome to vote”); } } // main method public static void main(String args[]) { try { // calling the method validate(13); } catch (InvalidAgeException ex) { System.out.println(“Caught the exception”); // printing the message from InvalidAgeException object System.out.println(“Exception occured: ” + ex); } System.out.println(“rest of the code…”); } } |
Output:

9. Difference between NoClassDefFoundError vs ClassNotFoundExcepiton in Java?
Both NoClassDefFoundError and ClassNotFoundException are dangerous errors that occur when the JVM or ClassLoader cannot find the class during the class loading process. This issue can also be caused by an incorrect CLASSPATH, as different ClassLoaders load classes from different locations. That is, some JAR files in lib are missing or older. They look very similar, but there are subtle differences between NoClassDefFoundError and ClassNotFoundException. NoClassDefFoundError indicates that the class existed at compile time but cannot be used at run time in a Java program. An error in the static initialization block can also result in a NoClassDefFoundError.
On the other hand, ClassNotFoundException has nothing to do with compile time. When I try to load a class at runtime using reflection, I get a ClassNotFoundException. Therefore, When loading the SQL driver, the corresponding class loader cannot find this class.
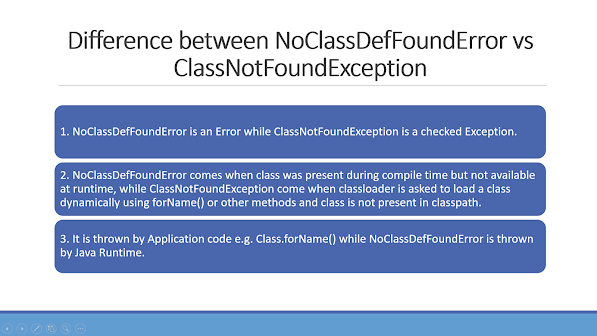
10. Can we have a try block without a catch block in Java?
Obviously yes, you can use the final block to create a try block without a catch block.
As you know, the final block is always executed, except for System.exit (), even if they try block raises an exception.
For Example
public class TryBlockWithoutCatch { public static void main(String[] args) { try { System.out.println(“Try Block”); } finally { System.out.println(“Finally Block”); } } } |
Output
Try Block Finally Block |
The final block is always executed, even if the method has a return type and the try block returns a value.