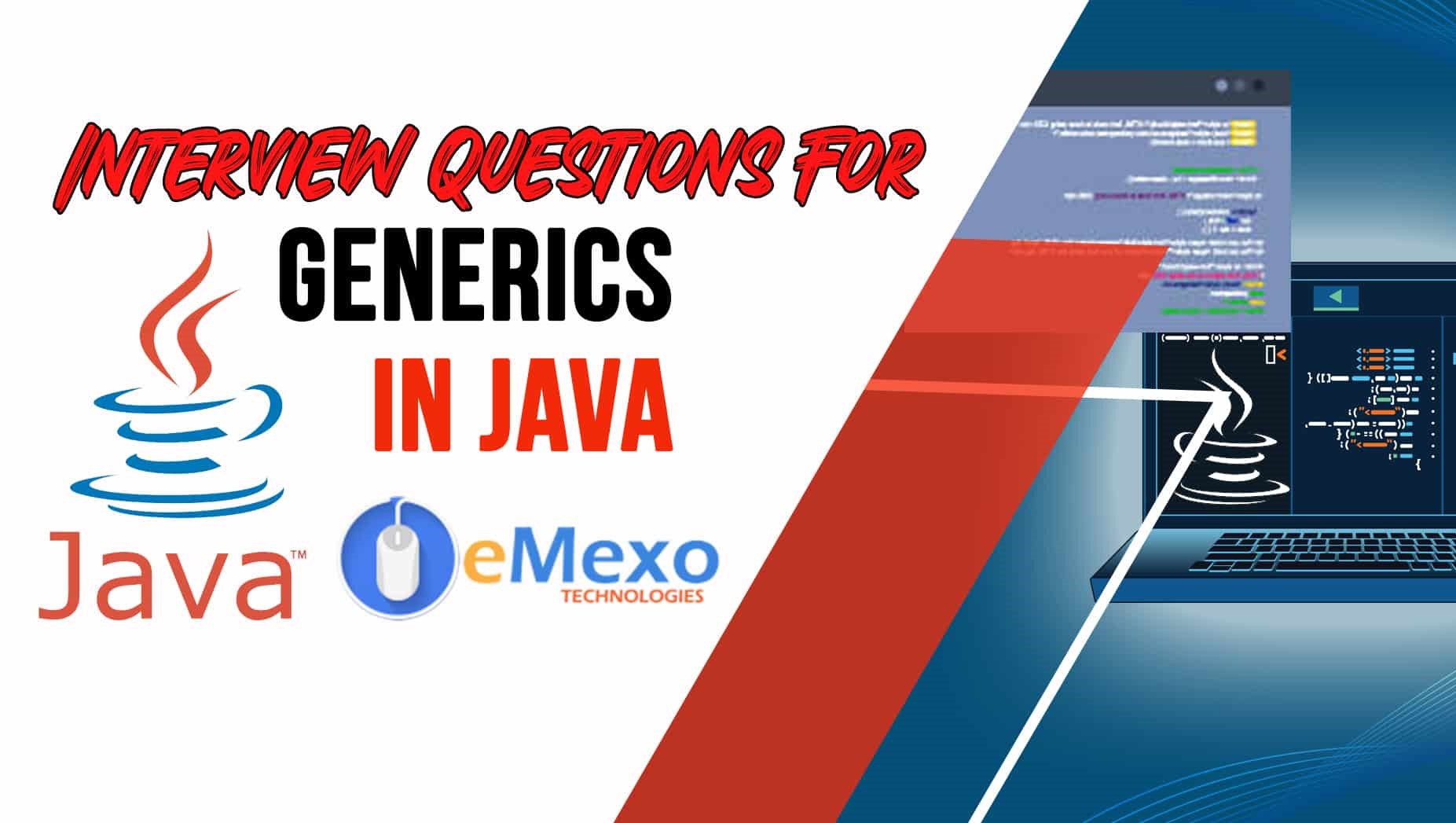
This is an article about the top 10 Java generics interview questions for both fresh and experienced candidates to help you get started with your dream job.
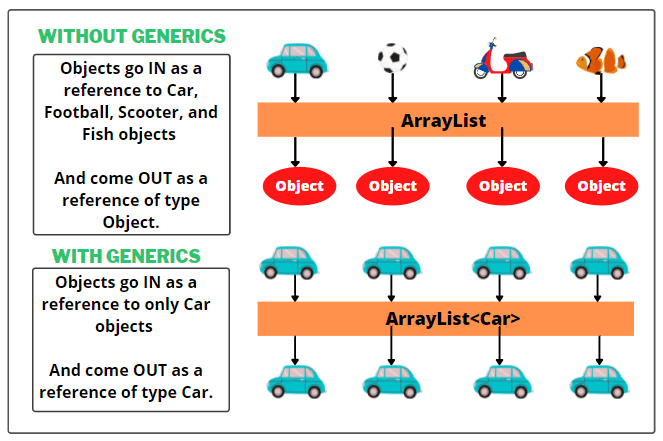
1. What is Generics in Java?
Java Generics are a set of related methods or similar types. Generics allow you to pass an integer, string, or user-defined type as a parameter to a class, method, or interface. Generics are primarily used in classes such as HashSet and HashMap.
2. What are the advantages of using generics?
- Generics guarantee compile-time safety. This allows the programmer to catch invalid types when compiling the code.
- Java Generics help programmers reuse any type of code. For example, a programmer creates a generic method for sorting an array of objects. With generics, programmers can use the same method for integer arrays, double arrays, and even string arrays. Another advantage of using
- generics is that you don’t need a separate typecast. The programmer defines the initial type and then leaves the work to the code.
- This allows you to implement uncommon algorithms.
3. What are the Types of Java Generics?
- Generic method:
- A generic Java method takes a parameter and returns a value after executing the task. It’s the same as a regular function, except that the generic method has type parameters quoted from the actual type. This makes generic methods more commonly available. The compiler provides security for programmers to easily code because the programmer does not have to do long individual typecasts.
- Generic Classes:
- Generic classes are implemented just like non-generic classes. The only difference is that it includes a type parameter section. You can specify multiple parameter types, separated by commas. A class that accepts one or more parameters is called a parameterized class or a parameterized type.
Example:
Single generic method
This example shows how to print different types of arrays using a single generic method.
class GenericMethodTest { // generic method printArray public static < E > void printArray( E[] inputArray ) { // Display array elements for(E element : inputArray) { System.out.printf(“%s “, element); } System.out.println(); } public static void main(String args[]) { // Create arrays of Integer, Double and Character Integer[] integerArray = { 5, 4, 3, 2, 1 }; Double[] doubleArray = { 1.21, 22.12, 13.32 }; Character[] characterArray = { ‘Y’, ‘o’, ‘u’, ‘ ‘, ‘a’, ‘r’, ‘e’, ‘ ‘, ‘a’,’w’,’e’,’s’,’o’,’m’,’e’ }; System.out.println(“integerArray contains:”); printArray(integerArray); // pass an Integer array System.out.println(“\ndoubleArray contains:”); printArray(doubleArray); // pass a Double array System.out.println(“\n characterArray contains:”); printArray(characterArray); // pass a Character array } } |
Output:
integerArray contains: 5 4 3 2 1 doubleArray contains: 1.21 22.12 13.32 characterArray contains: Y o u a r e a w e s o m e |
Generic class
This example shows how to define a generic class:
class container<T> { private T obj1; public void add(T obj1) { this.obj1 = obj1; } public T get() { return obj1; } public static void main(String[] args) { container<Integer> integercontainer= new container<Integer>(); container<String> stringcontainer = new container<String>(); integercontainer.add(new Integer(7)); stringcontainer.add(new String(“You are awesome”)); System.out.printf(“Integer Value :%d\n\n”, integercontainer.get()); System.out.printf(“String Value :%s\n”, stringcontainer.get()); } } |
Output:
Integer Value : 7 String Value : You are awesome |
4. How can you suppress the unchecked warnings in Java?
The Javac compiler for Java 5 produces unchecked warnings when mixing raw and generic types. For example:
List<String> rawList = new ArrayList() Note: Hello.java uses unchecked or unsafe operations.; |
This can be suppressed with the @SuppressWarnings(“unchecked”) annotation.
5. What Is Type Inference?
Type inference is when the compiler can infer the generic type by looking at the type of the method argument. For example, if you pass T to a method that returns T, the compiler can determine the return type. Let’s try this by calling the generic method from the previous question.
Integer inferredInteger = returnType(1); String inferredString = returnType(“String”); |
As you can see, no cast is required and no generic type arguments need to be passed. The argument type only infers the return type.
6. How to write parametrized class in Java using Generics?
This is an extension of the previous Java Generic Interview question. Instead of asking you to create a generic method, the interviewer can ask you to create a type-safe class using generics. Again, it’s important to note that instead of using the raw type, you should use the generic type and always use the default wildcards used by the JDK.
7. Can we use Generics with Array?
At first, If you know the fact that Array doesn’t support Generics, this was probably the simplest interview question about Generics in Java. That Effective Java prioritizes lists over arrays because they can provide type safety over arrays during compilation.
8. What Is a Wildcard Type?
Wildcard types represent unknown types. You will see a question mark similar to the following:
public static void consumeListOfWildcardType(List<?> list) |
At last, Here is a list of any kind. Thus you can pass any list to this method.
9. Is It Possible to Declared a Multiple Bounded Type Parameter?
It is possible to declare multiple boundaries with a generic type. In the previous example, you specified a single boundary, but you can specify more if you prefer.
public abstract class Cage<T extends Animal & Comparable> |
In this example, the animal is a class, as well as an interface. In addition, now our type needs to respect these two ceilings. If our type was a subclass of animals but didn’t implement an equivalent, the code wouldn’t compile. Also note that if one of the upper bounds is a class, it must be the first argument.
10. How Does a Generic Method Differ from a Generic Type?
A generic method introduces a type parameter to a method that is within the scope of that method. Let’s try an example:
public static <T> T returnType(T argument) { return argument; } |
I used a static method, but you can also use a non-static method if you prefer. Finally, By using type inference (discussed in the next question), you can call it just like a regular method, without specifying a type argument.
Last but not least, start your career at Dream Company at eMexo Technologies, enroll in Java training in Electronic City Bangalore, obtain Java certification, and start your career at Dream Company. |