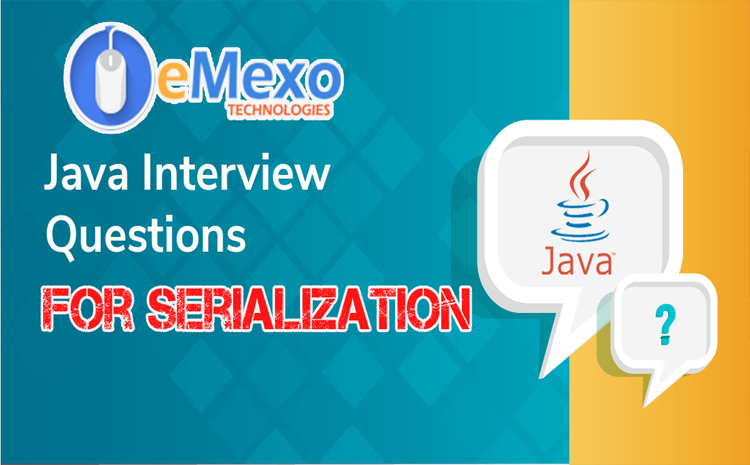
Serialization is an important topic in Java interviews. Java developers need to know the answer to the serialization interview questions.
In this article, we’ll look at the top Java Serialization Interview Questions with Answers. Covered questions for both beginners and experienced professionals.
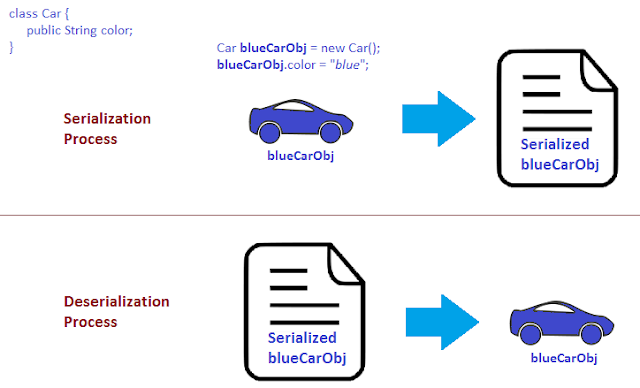
Take this premier Java training in Electronic City Bangalore to learn about Java Programming. After successfully completing this Java certification training in eMexo Technologies, candidates can easily pass the certification exam. The average salary for Java practitioners is $ 73,743 per year. Don’t think twice, join us today.
1. What’s serialization in java?
- Serializing an object in Java is the process of converting the object to binary format, which can be stored on a disk or sent over the network to other running Java virtual machines.
- Java provides a serialization API for serializing and deserializing objects such as java.io.Serializable, java.io.Externalizable, ObjectInputStream, and ObjectOutputStream.
- Java programmers are free to use the standard Java-based serialization mechanism. Although it is a class, you are free to use your own custom binary format. This is recommended as a serialization best practice as the serialized binary format will be part of the class’s exported API and the private and package-private field formats can break encapsulation. Often. It is provided in Java.
2. How to make a java class Serializable?
- Making a class Serializable in Java is very easy, Your Java class just needs to implement java.io.Serializable interface and JVM will take care of serializing objects in the default format.
- The decision to make a Class Serializable should be taken concisely because though near term cost of making a Class Serializable is low, the long-term cost is substantial and it can potentially limit your ability to further modify and change its implementation because like any public API, serialized form of an object becomes part of public API and when you change the structure of your class by implementing addition interface, adding or removing any field can potentially break default serialization, this can be minimized by using a custom binary format but still requires a lot of effort to ensure backward compatibility.
- One example of How Serialization can put constraints on your ability to change class is SerialVersionUID.
- If you don`t explicitly declare SerialVersionUID then JVM generates it based upon the structure of the class which depends upon the interfaces a class implements and several other factors which are subject to change.
- Suppose you implement another interface then JVM will generate a different SerialVersionUID for a new version of class files and when you try to load an old object serialized by the old version of your program you will get InvalidClassException.
3. Difference between transient and volatile in Java?
- Volatile and transient are two completely different keywords from different areas of the Java programming language.
- The keyword transient is used during the serialization of Java objects, while volatile refers to the visibility of variables modified by multiple threads during concurrent programming.
- The only similarity between volatile and transient is that they are less commonly used or uncommon keywords and are not as popular as public, static, or final.
- The transient keyword is used with instance variables and is excluded from the serialization process.
- If a field is temporary, its value is not maintained. On the other hand, you can also use the volatile keyword on a variable to specify a compiler and JVM that always read the value from main memory and follow the pre-occurrence relationship of volatile variable visibility across multiple threads.
- The transient keyword cannot be used with the static keyword, but the volatile can be used with static.
- Temporary variables are initialized with default values during deserialization, and value assignment or restoration must be handled by the application code.
4. What’s a transient variable in Java?
- One-Word transients use keywords in the serialization process to prevent variables from being serialized. So if you have a field that doesn’t make sense to serialize, just declare it as temporary and it won’t be serialized.
- We know the purpose of temporary keywords or variables. It makes sense to think about which variables need to be marked as temporary. My rule doesn’t need to store a variable whose value can be calculated from other variables.
- For example, if you have a field called “Interest” whose value can be obtained from other fields such as the following, in principle, rates, times, etc., you don’t need to serialize.
- Another example is the number of words. If you save the article, you don’t need to save the word count because you can create it when the article is deserialized.
- Another good example of an ephemeral keyword is “logger”. Most of the time I have a logger instance to log in to Java, but do I need to serialize it properly?
For Example:
public class Stock { private transient Logger logger = Logger.getLogger(Stock.class); //will not serialized private String symbol; //will be serialized private BigInteger price; //serialized private long quantity; //serialized } |
- Transient keywords can only be applied to fields or member variables. Applying it to a method or local variable will result in a compilation error.
- Another important point is that you can declare variables static and transient at the same time, and the Java compiler won’t complain, but transient tells the statement “Don’t save this field”, and static variables do. It doesn’t make sense because it’s not. It is not saved during serialization anyway.
- Similarly, you can apply the transient and final keywords together to the variable compiler. This won’t complain, but it faces another problem of reinitializing the final variable during deserialization.
- Java transient variables are not persisted or saved when the object is serialized.
5. Difference between Serializable and externalizable in Java?
- Both serializable and extensible are used to serialize or persist Java objects, but there is no big difference in how they are done.
- For Serializable, the Java Virtual Machine has full control over object serialization, while for Externalizable, the application controls persistent objects.
- The writeExternal () and readExternal () methods provide an application that gives you complete control over the format and content of the serialization process.
- You can use it to improve the performance and speed of the serialization process.
- For Serializable, the standard serialization process is used.
- For Externalizable, the custom serialization process implemented by the application is used.
- The JVM calls readExternal () and writeExternal () on the java.io.Externalizalbe interface to restore the object and write it to persistence.
- The external interface gives you complete control over the application serialization process. readExternal () and writeExternal () replace specific implementations of the writeObject and readObject methods.
- Externalizable provides writeExternal () and readExternal () methods that give you the flexibility to control Java’s serialization mechanism instead of relying on Java’s default serialization.
- Proper implementation of an externalizable interface can significantly improve the performance of your application.
6. How many methods does Serializable have? If no method then what is the purpose of the Serializable interface?
- Serializable interfaces reside in the java.io package and form the core of the Java serialization mechanism. It lacks the
- method and is also known as the marker interface in Java.
- If the class implements the java.io.Serializable interface, the class will be serializable in Java, indicating to the compiler that the Java serialization mechanism is being used to serialize this object.
7. What is serialVersionUID? What would happen if you don’t define this?
- The SerialVersionUID is the ID that is stamped on the object when it is serialized, usually the hash code of the object. You can use the serialver tool to view the serialVersionUID of a serialized object.
- The SerialVersionUID is used for object versioning. You can also specify the serialVersionUID in the
- class file. If you do not specify
- serialVersionUID, you will not be able to restore an already serialized class because the serialVersionUID generated for the new class and the old serialized object will be different when you add or modify fields in the class.
- The Java serialization process relies on the correct serialVersionUID to restore the state of the serialized object and throw a java.io.InvalidClassException if the serialVersionUID does not match.
8. While serializing you want some of the members not to serialize? How do you achieve it?
If you don’t want the field to be part of the object state, declare it as static or temporary as needed. That way, you won’t be part of the Java serialization process.
9. What will happen if one of the members of the class doesn’t implement a Serializable interface?
If you try to serialize an object in a class that implements Serializable, but the object contains a reference to a non-serializable class, a “NotSerializableException
” is thrown at run time, so the code always has a SerializableAlert (comment section in my code). To Instruct the developer to remember this fact when adding a new field to a serializable class.
10. If a class is Serializable but its superclass is not, what will be the state of the instance variables inherited from the super class after deserialization?
- The Java serialization process simply moves up the object hierarchy until the class is serializable. Because of this, it implements a Serializable interface in Java and initializes instance variables by calling the non-serializable superclass constructor during deserialization.
- Once the constructor chain is started, it cannot be stopped. Therefore, the constructor is executed even if the class above the hierarchy implements a Serializable interface.
11. Can you Customize the Serialization process or can you override the default Serialization process in Java?
- The answer is yes, you can.
- Everyone knows that ObjectOutputStream.writeObject (saveThisobject) is called to serialize an object and ObjectInputStream.readObject () is called to read an object, but there is another Java virtual machine, Provides these definitions.
- There are two methods in the class. If you define these two methods in the class, the JVM will call these two methods instead of using the default serialization mechanism.
- You can customize the serialization and deserialization behavior of objects here by performing any type of pre-or post-processing task.
- An important caveat is to keep these methods private to avoid inheritance, overrides, or overloads.
- Only Java virtual machines can call private methods, so class integrity is maintained and Java serialization works fine.
12. Suppose the superclass of a new class implements a Serializable interface, how can you avoid the new class being serialized?
- If the class’s superclass already implements a Java-serializable interface, it is already Java-serializable. It’s not really possible to make a non-serializable class because the interface can’t be unimplemented, but there is a way to do so. Avoid serializing new classes.
- To avoid Java serialization, you need to implement the writeObject () and readObject () methods in your class and throw a NotSerializableException from these methods.
13. Which methods are used during the Serialization and Deserialization process in Java?
- Java serialization is performed by the java.io.ObjectOutputStream class.
- This class is a filter stream that wraps around a lower-level byte stream to handle the serialization mechanism.
- Call ObjectOutputStream.writeObject (saveThisobject) to save the object through the serialization mechanism, and call the ObjectInputStream.readObject () method to deserialize this object.
- Calling the writeObject () method triggers the serialization process in Java.
- One of the important things to note about the readObject () method is that it is used to read bytes from persistence, create an object from those bytes, and return an object that is cast to the correct type.
14. If you serialize and store a class in persistence, you might update that class to add a new field. What will happen if you deserialize the object already serialized?
- It depends on whether the class has its own serialVersionUID.
- If you do not specify a serialVersionUID in your code, it is generated by the Java compiler and is usually the same as the object’s HashCode.
- If you add a new field, the new serialVersionUID generated for this class version may not be the same as an object that has already been serialized.
- In that case, the Java serialization API throws a java.io.InvalidClassException. For this reason, it’s a good idea to include your own serialVersionUID in your code so that it’s always the same in a single class.
15. What are the compatible changes and incompatible changes in Java Serialization Mechanism?
- The real challenge is to change the class structure by adding fields and methods or removing fields and methods that have objects that are already serialized.
- According to the Java serialization specification, adding a field or method is a compliant change, and changing the class hierarchy or UN implementation of a serializable interface is partly a non-compliant change.
16. Can we transfer a Serialized object via network?
Yes, Serialized Java objects remain in the form of bytes that can be sent over the network, so you can send serialized objects over the network. You can also save the serialized object as a blob on a disk or database.
17. Which kind of variables is not serialized during Java Serialization?
- Because static variables belong to a class rather than an object, they are not part of the object’s state and are not saved during the Java serialization process. This is because Java serialization only preserves the state of the object, not the object itself.
- Transient variables are also not part of the Java serialization process and are not part of the object’s serialization state.